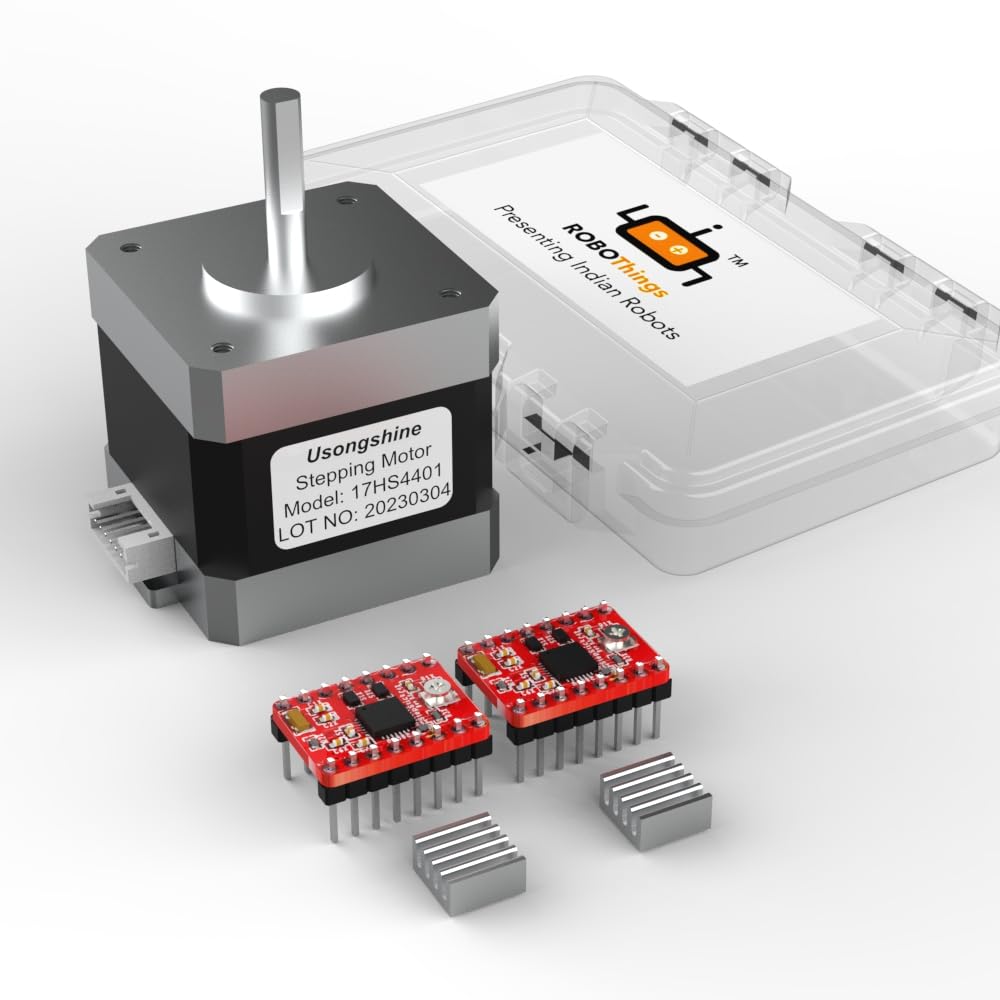
Product Overview:
Welcome to RoboThings! Today, we’ll walk you through using the A4988 stepper motor driver with Arduino IDE for the first time. This guide is perfect for beginners and will help you control a stepper motor with precision. Let’s dive in!
What You’ll Need
- Arduino board (e.g., Uno, Mega, Nano)
- A4988 stepper motor driver
- Stepper motor
- Breadboard and jumper wires
- Power supply (appropriate for your stepper motor)
- Capacitors (optional, for noise reduction)
- Resistors (optional, for current limiting)
Step 1: Install the Arduino IDE
If you haven’t already, download and install the Arduino IDE from the official Arduino website.
2: Setting Up the Hardware
Connecting the A4988 Driver to the Arduino
First, let’s connect the A4988 driver to the Arduino. The A4988 has several pins that need to be connected:
- VMOT and GND: Connect VMOT to the motor power supply and GND to the ground of the power supply. It’s a good idea to add a capacitor (e.g., 100µF) across VMOT and GND to reduce noise.
- VDD and GND: Connect VDD to the 5V pin on the Arduino and GND to the Arduino’s ground.
- 2B, 2A, 1A, 1B: Connect these to the stepper motor coils. The order depends on your motor’s datasheet.
- STEP and DIR: Connect these to two digital pins on the Arduino (e.g., 2 and 3).
- EN: This pin can be used to enable/disable the driver. Connect to a digital pin on the Arduino if you want to control it programmatically, otherwise, connect it to GND.
- MS1, MS2, MS3: These pins control the microstepping settings. You can leave them unconnected for full-step mode or connect them to GND or VDD for different microstepping modes.
Wiring Diagram
Here’s a simple wiring diagram to illustrate the connections:
Step 3: Programming the Arduino
Now that the hardware is set up and the library is installed, let’s write some code to control the stepper motor.
Sample Code
Here’s a simple example to get you started:
#include
// Define the pins for the stepper motor
#define DIR_PIN 2
#define STEP_PIN 3
// Create an instance of the AccelStepper class
AccelStepper stepper(AccelStepper::DRIVER, STEP_PIN, DIR_PIN);
void setup() {
// Set the maximum speed and acceleration
stepper.setMaxSpeed(1000);
stepper.setAcceleration(500);
}
void loop() {
// Move the motor 200 steps in one direction
stepper.moveTo(200);
stepper.runToPosition();
delay(1000); // Wait for a second
// Move the motor back to the original position
stepper.moveTo(0);
stepper.runToPosition();
delay(1000); // Wait for a second
}
Explanation
- We include the AccelStepper library.
- Define the pins connected to the STEP and DIR pins on the A4988.
- Create an instance of the AccelStepper class.
- In the
setup
function, we set the maximum speed and acceleration. - In the
loop
function, we move the motor 200 steps in one direction and then back to the original position with a delay in between.
Step 4: Uploading and Testing
- Connect your Arduino to your computer via USB.
- Select the correct board and port from the Tools menu.
- Click the Upload button.
Once the code is uploaded, you should see your stepper motor moving back and forth. If it doesn’t work, double-check your wiring and ensure that your motor and driver are getting the correct voltage.
Conclusion
You’ve successfully connected and controlled a stepper motor using the A4988 driver and Arduino IDE. This setup allows for precise control of stepper motors, which is essential for many robotics and CNC projects. Feel free to experiment with different speeds, accelerations, and microstepping settings to see how they affect the motor’s behavior.
Stay tuned for more tutorials and project ideas here on RoboThings! If you have any questions or run into issues, leave a comment below, and we’ll be happy to help.
Happy making!
Note: This blog post assumes basic familiarity with Arduino IDE. If you’re completely new to Arduino, you might want to check out our beginner’s guide first.
Feel free to ask if you need additional customization or have specific requirements for this blog post.